简介
总结下之前看的自定义View的内容,结合一个简单的例子,阐述下基本用法和大致的使用流程,这个例子比较简单,更复杂的自定义View,随着自己的学习,后面再慢慢添加。作为一个Android开发者,这部分应该是不可或缺的。
自定义属性
位置:res/values/attrs.xml
格式:
1 2 3 4 5 6
| <?xml version="1.0" encoding="utf-8"?> <resources> <declare-styleable name="name_of_style"> <attr name="name_of_attr" format="reference|string|color|boolean|dimension|enum|flag|float|fraction|integer"/> </declare-styleable> </resources>
|
format |
意义 |
reference |
参考某一资源ID, 如R.drawable.xxx |
string |
字符串 |
color |
颜色 |
boolean |
布尔值 |
dimension |
尺寸值 |
enum |
枚举值 |
flag |
位或运算 |
float |
浮点数 |
fraction |
百分数,例如pivotX,pivotY这一类属性 |
integer |
整数 |
获取自定义属性
1 2 3 4
| TypedArray typedArray = context.obtainStyledAttributes(attrs, R.styleable.name_of_style);
Color mColor = typedArray.getColor(R.styleable.name_of_style_name_of_attr, Color.BLUE); typedArray.recycle();
|
名字: R.styleable.{name_of_style} R.styleable.{name_of_style}_{name_of_attr}
举例说明
举个例子,实现一个背景为渐变色的圆角按钮,圆角半径,开始颜色,中心颜色,结束颜色,渐变方向用户可自定义。
attrs.xml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| <?xml version="1.0" encoding="utf-8"?> <resources> <declare-styleable name="CornerButton"> <attr name="corner_radius" format="dimension" /> <attr name="background_start_color" format="color" /> <attr name="background_center_color" format="color" /> <attr name="background_end_color" format="color" /> <attr name="backgrouund_gradient_orientation"> <enum name="TOP_BOTTOM" value="0" /> <enum name="TR_BL" value="1" /> <enum name="RIGHT_LEFT" value="2" /> <enum name="BR_TL" value="3" /> <enum name="BOTTOM_TOP" value="4" /> <enum name="BL_TR" value="5" /> <enum name="LEFT_RIGHT" value="6" /> <enum name="TL_BR" value="7" /> </attr> </declare-styleable> </resources>
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54
| public class CornerButton extends Button {
private GradientDrawable mBg; private float mRandius; private int mStartColor; private int mCenterColor; private int mEndColor; private int mOrientation;
public CornerButton(Context context, AttributeSet attrs) { super(context, attrs);
TypedArray typedArray = context.obtainStyledAttributes(attrs, R.styleable.CornerButton); mRandius = typedArray.getDimension(R.styleable.CornerButton_corner_radius, 10); mStartColor = typedArray.getColor(R.styleable.CornerButton_background_start_color, Color.BLUE); mCenterColor = typedArray.getColor(R.styleable.CornerButton_background_center_color, Color.GREEN); mEndColor = typedArray.getColor(R.styleable.CornerButton_background_end_color, Color.BLACK); mOrientation = typedArray.getInt(R.styleable.CornerButton_backgrouund_gradient_orientation, 0); typedArray.recycle();
int[] colors = {mStartColor, mCenterColor, mEndColor}; mBg = new GradientDrawable(); mBg.setCornerRadius(mRandius); mBg.setOrientation(Orientation.TR_BL); mBg.setColors(colors); switch (mOrientation) { case 0: mBg.setOrientation(Orientation.TOP_BOTTOM); break; case 1: mBg.setOrientation(Orientation.TR_BL); break; case 2: mBg.setOrientation(Orientation.RIGHT_LEFT); break; case 3: mBg.setOrientation(Orientation.BR_TL); break; case 4: mBg.setOrientation(Orientation.BOTTOM_TOP); break; case 5: mBg.setOrientation(Orientation.BL_TR); break; case 6: mBg.setOrientation(Orientation.LEFT_RIGHT); break; case 7: mBg.setOrientation(Orientation.TL_BR); break; } this.setBackground(mBg); } }
|
activity_main.xml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| <?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:yidong="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:id="@+id/activity_main" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" tools:context="gs.com.customview.MainActivity">
<gs.com.customview.CornerButton android:id="@+id/cb_content" android:layout_width="match_parent" android:layout_height="match_parent" yidong:background_start_color="#CCFF0000" yidong:background_center_color="#CCAADD00" yidong:background_end_color="#CC00EEFF" yidong:corner_radius="100dp" yidong:backgrouund_gradient_orientation="BOTTOM_TOP" /> </RelativeLayout>
|
xmlns:yidong=“http://schemas.android.com/apk/res-auto”
和
yidong:corner_radius=“100dp”
XML命名空间和属性的Tag对应。
效果
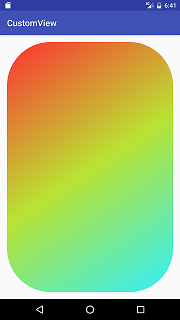
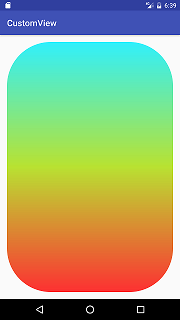